Python
To set up BAML with Python do the following:
Install BAML VSCode/Cursor Extension
https://marketplace.visualstudio.com/items?itemName=boundary.baml-extension
- syntax highlighting
- testing playground
- prompt previews
In your VSCode User Settings, highly recommend adding this to get better autocomplete for python in general, not just BAML.
Generate the baml_client python module from .baml files
One of the files in your baml_src
directory will have a generator block. The next commmand will auto-generate the baml_client
directory, which will have auto-generated python code to call your BAML functions.
Any types defined in .baml files will be converted into Pydantic models in the baml_client
directory.
See What is baml_client to learn more about how this works.
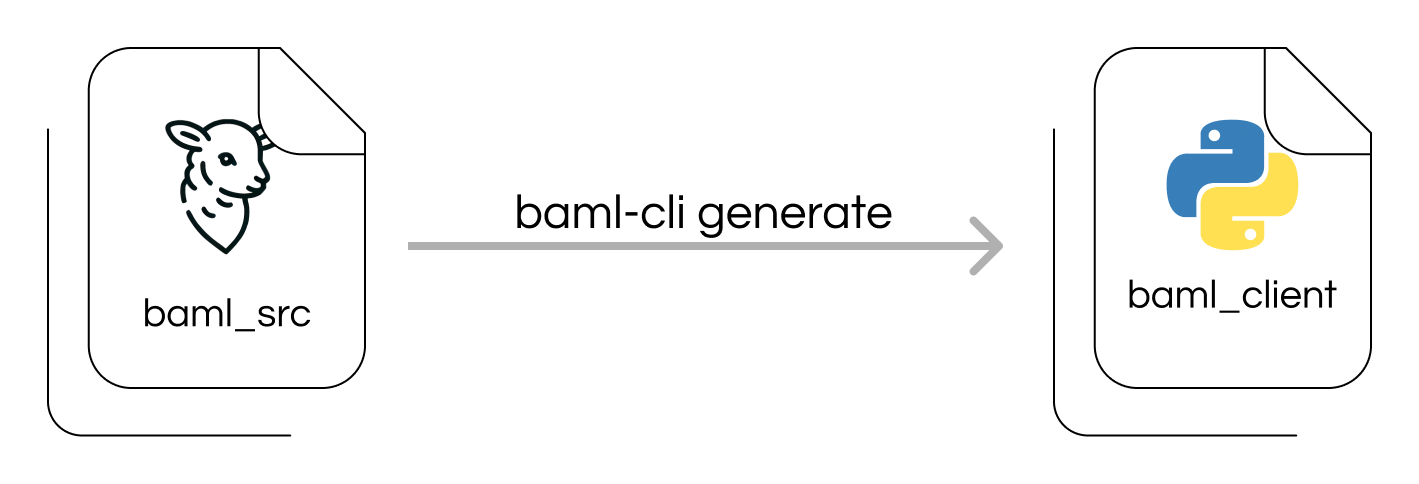
If you set up the VSCode extension, it will automatically run baml-cli generate
on saving a BAML file.
BAML with Jupyter Notebooks
You can use the baml_client in a Jupyter notebook.
One of the common problems is making sure your code changes are picked up by the notebook without having to restart the whole kernel (and re-run all the cells)
To make sure your changes in .baml files are reflected in your notebook you must do these steps:
Setup the autoreload extension
This will make sure to reload imports, such as baml_client’s “b” object before every cell runs.
Import baml_client module in your notebook
Note it’s different from how we import in python.
Usually we import things as
from baml_client import b
, and we can call our functions using b
, but the %autoreload
notebook extension does not work well with from...import
statements.
Call BAML functions using the module name as a prefix
Now your changes in .baml files are reflected in your notebook automatically, without needing to restart the Jupyter kernel.
If you want to keep using the from baml_client import b
style, you’ll just need to re-import it everytime you regenerate the baml_client.
Pylance will complain about any schema changes you make in .baml files. You can ignore these errors. If you want it to pick up your new types, you’ll need to restart the kernel. This auto-reload approach works best if you’re only making changes to the prompts.
You’re all set! Continue on to the Deployment Guides for your language to learn how to deploy your BAML code or check out the Interactive Examples to see more examples.