Ruby
You can check out this repo: https://github.com/BoundaryML/baml-examples/tree/main/ruby-starter
To set up BAML with Ruby do the following:
Install BAML VSCode Extension
https://marketplace.visualstudio.com/items?itemName=boundary.baml-extension
- syntax highlighting
- testing playground
- prompt previews
Generate Ruby code from .baml files
` See What is baml_src to learn more about how this works.
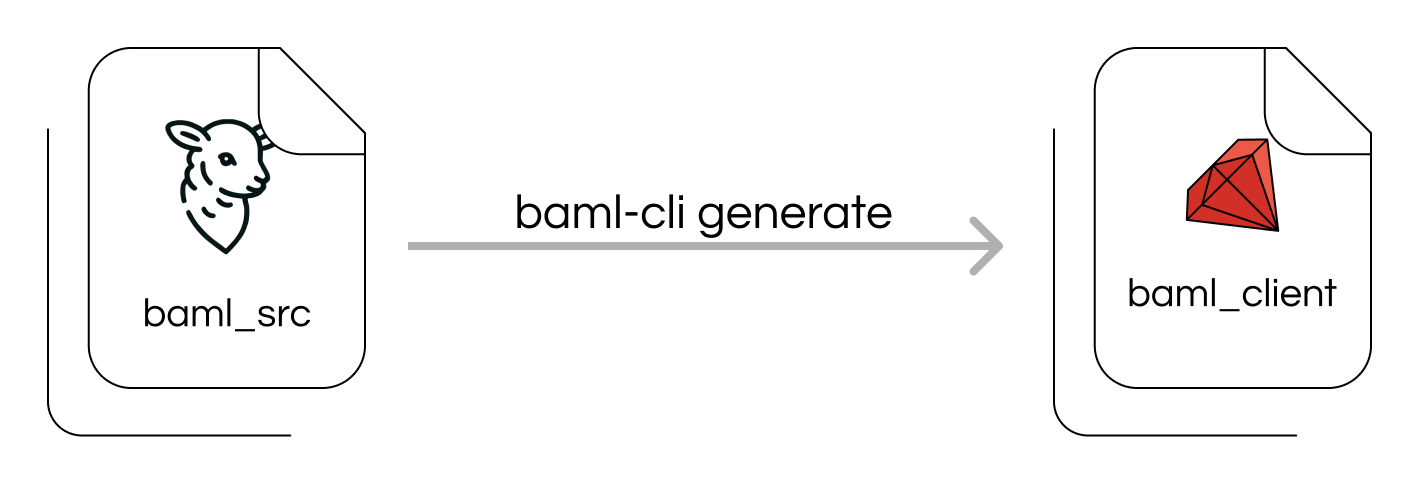
As fun as writing BAML is, we want you be able to leverage BAML with existing ruby modules. This command gives you a ruby module that is a type-safe interface to every BAML function.
Our VSCode extension automatically runs this command when you save a BAML file.