anthropic
The anthropic
provider supports all APIs that use the same interface for the /v1/messages
endpoint.
Example:
The options are passed through directly to the API, barring a few. Here’s a shorthand of the options:
Non-forwarded options
Will be passed as a bearer token. Default: env.ANTHROPIC_API_KEY
Authorization: Bearer $api_key
The base URL for the API. Default: https://api.anthropic.com
The default role for any prompts that don’t specify a role. Default: system
We don’t have any checks for this field, you can pass any string you wish.
Additional headers to send with the request.
Unless specified with a different value, we inject in the following headers:
Example:
Which role metadata should we forward to the API? Default: []
For example you can set this to ["cache_control"]
to forward the cache policy to the API.
If you do not set allowed_role_metadata
, we will not forward any role metadata to the API even if it is set in the prompt.
Then in your prompt you can use something like:
You can use the playground to see the raw curl request to see what is being sent to the API.
Forwarded options
BAML will auto construct this field for you from the prompt, if necessary.
Only the first system message will be used, all subsequent ones will be cast to the assistant
role.
BAML will auto construct this field for you from the prompt
BAML will auto construct this field for you based on how you call the client in your code
The model to use.
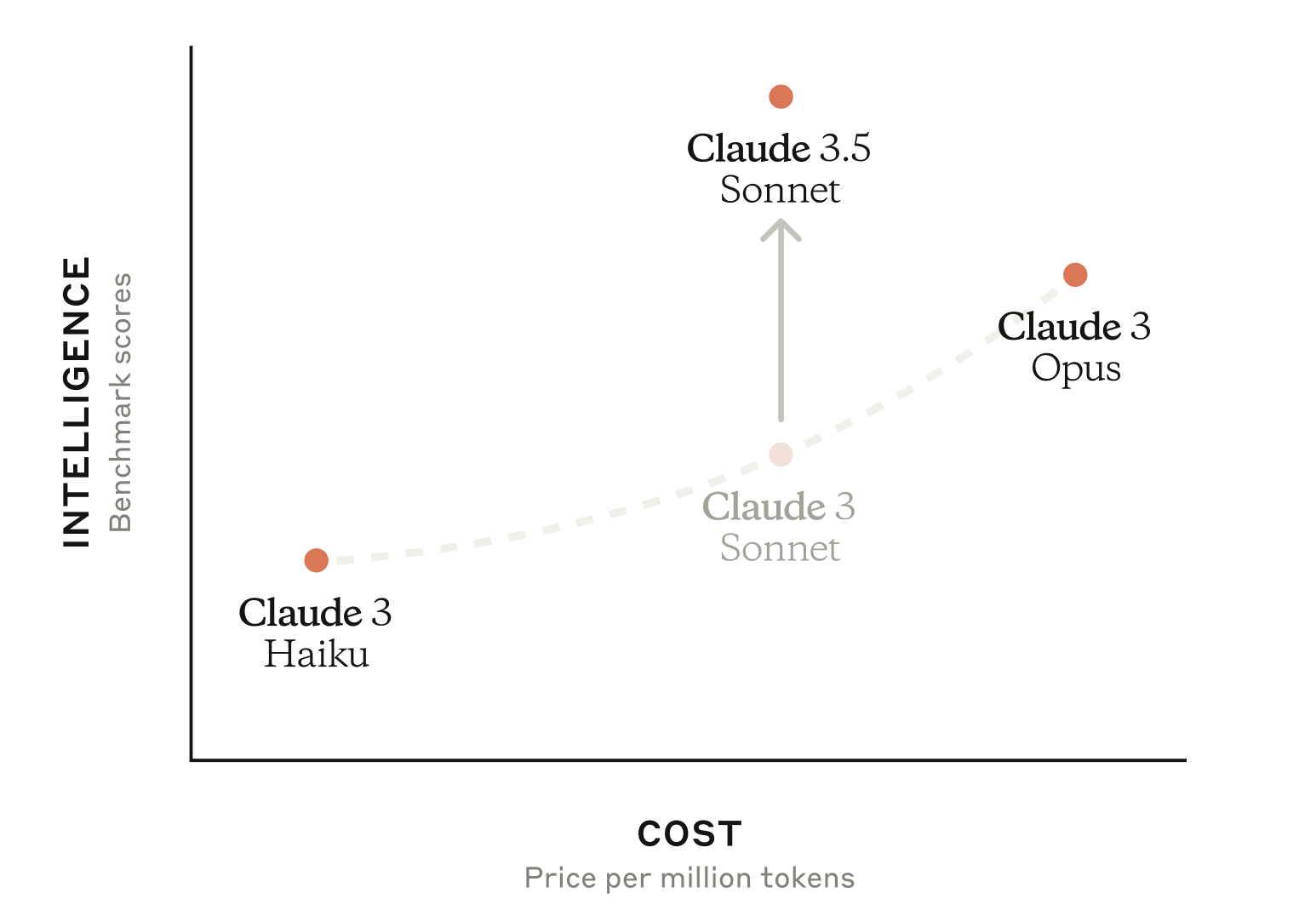
See anthropic docs for the latest list of all models. You can pass any model name you wish, we will not check if it exists.
The maximum number of tokens to generate. Default: 4069
For all other options, see the official anthropic API documentation.