Extracting Action Items from Meeting Transcripts
In this tutorial, you’ll learn how to build a BAML function that automatically extracts structured action items from meeting transcripts. By the end, you’ll have a working system that can identify tasks, assignees, priorities, subtasks, and dependencies.
Prerequisites
- Basic understanding of BAML syntax
- An OpenAI API key configured in your environment
Step 1: Define the Data Models
First, let’s define the data structures for our tasks. Create a new BAML file called action_items.baml
and add these class definitions:
These models define:
- A
Subtask
class for breaking down larger tasks - A
Priority
enum for task urgency levels - A
Ticket
class that represents a complete task with all its metadata
Step 2: Create the Task Extraction Function
Next, we’ll create a function that uses GPT-4 to analyze meeting transcripts and extract tasks:
This function:
- Takes a meeting transcript as input
- Returns an array of
Ticket
objects - Uses GPT-4 to analyze the transcript
- Includes clear instructions in the prompt for task extraction
Step 3: Test the Implementation
Let’s add test cases to verify our implementation works correctly. Add these test cases to your BAML file:
These tests provide:
- A simple case with a single task and subtask
- A complex case with multiple tasks, priorities, dependencies, and assignees
This is what you see in the BAML playground:
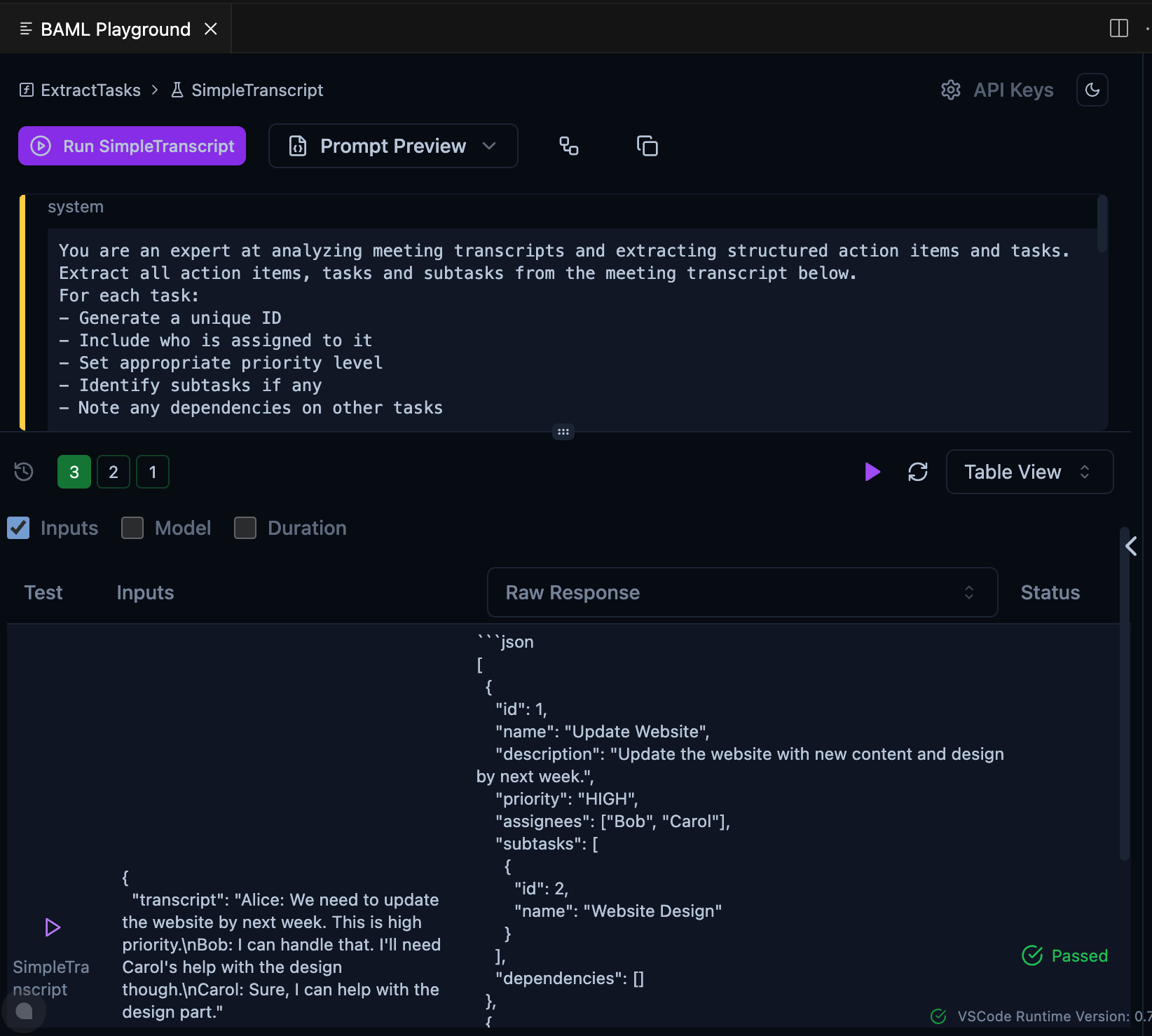
This is the output from the complex test case:
What’s Next?
You can enhance this implementation by:
- Adding due dates to the
Ticket
class - Including status tracking for tasks
- Adding validation for task dependencies
- Implementing custom formatting for the extracted tasks
Common Issues and Solutions
- If tasks aren’t being properly identified, try adjusting the prompt to be more specific
- If priorities aren’t being set correctly, consider adding examples in the prompt
- For complex transcripts, you might need to adjust the model parameters for better results