aws-bedrock
AWS Bedrock provider for BAML
The aws-bedrock
provider supports all text-output models available via the
Converse
API.
Example:
Authorization
We use the AWS SDK under the hood, which will respect all authentication mechanisms supported by the SDK, including but not limited to:
- loading the specified
AWS_PROFILE
from~/.aws/config
- built-in authn for services running in EC2, ECS, Lambda, etc.
- You can also specify the access key ID, secret access key, and region directly (see below)
Playground setup
Add these three environment variables to your extension variables to use the AWS Bedrock provider in the playground.
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
AWS_REGION
- likeus-east-1
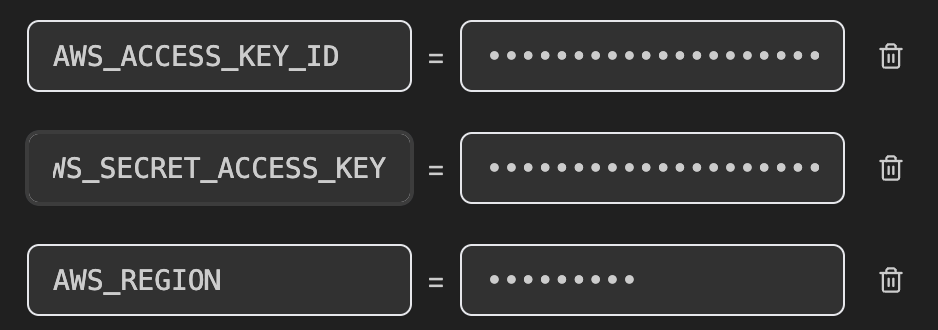
Non-forwarded options
The default role for any prompts that don’t specify a role. Default: system
We don’t have any checks for this field, you can pass any string you wish.
The role to use if the role is not in the allowed_roles. Default: "user"
usually, but some models like OpenAI’s gpt-4o
will use "system"
Picked the first role in allowed_roles
if not “user”, otherwise “user”.
Which roles should we forward to the API? Default: ["system", "user", "assistant"]
usually, but some models like OpenAI’s o1-mini
will use ["user", "assistant"]
When building prompts, any role not in this list will be set to the default_role
.
Which role metadata should we forward to the API? Default: []
For example you can set this to ["foo", "bar"]
to forward the cache policy to the API.
If you do not set allowed_role_metadata
, we will not forward any role metadata to the API even if it is set in the prompt.
Then in your prompt you can use something like:
You can use the playground to see the raw curl request to see what is being sent to the API.
Whether the internal LLM client should use the streaming API. Default: true
Then in your prompt you can use something like:
The AWS region to use. Default: AWS_REGION
environment variable
We don’t have any checks for this field, you can pass any string you wish.
The AWS access key ID to use. Default: AWS_ACCESS_KEY_ID
environment variable
The AWS secret access key to use. Default: AWS_SECRET_ACCESS_KEY
environment variable
Forwarded options
BAML will auto construct this field for you from the prompt
The model to use.
Run aws bedrock list-foundation-models | jq '.modelSummaries.[].modelId
to get
a list of available foundation models; you can also use any custom models you’ve
deployed.
Note that to use any of these models you’ll need to request model access.
Additional inference configuration to send with the request; see AWS Bedrock documentation.
Example: